How to Install and Manage PostGIS with a Non-Superuser Role
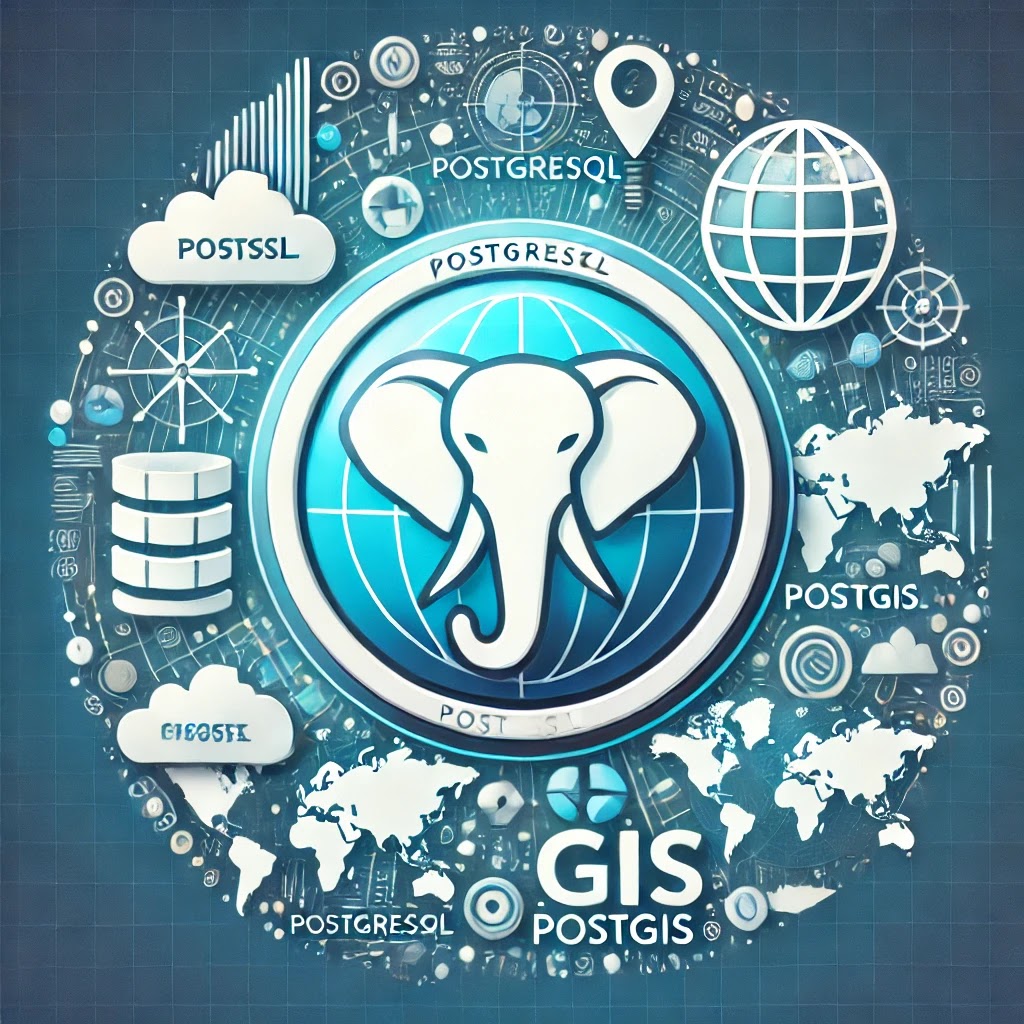
In the ever-evolving world of Python development, managing project dependencies and Python versions can become a complex task. Tools like Pipenv and Pyenv have been created to simplify these processes, but understanding how they work individually and together is crucial for efficient project management. This comprehensive guide will delve into the functionalities of Pipenv and Pyenv, highlight their differences, and demonstrate how to effectively use them in your Python projects.
Pipenv is a tool designed to bring the best of all packaging worlds to Python developers, combining functionality from pip
, virtualenv
, and more. It aims to simplify the management of dependencies and virtual environments in Python projects.
Pipfile
, tracking package versions and ensuring consistency.Pipfile.lock
file to lock dependencies to specific versions, ensuring deterministic builds across different systems.Pipfile
and Pipfile.lock
accordingly.Here are some common Pipenv commands to manage your Python projects:
Pipfile
.
pipenv install
Pipfile
.
pipenv install package_name
pipenv install --dev
Pipfile
.
pipenv uninstall package_name
pipenv shell
pipenv run python script.py
pipenv check
pipenv update
requirements.txt
file from the Pipfile.lock
.
pipenv lock -r > requirements.txt
Pipfile.lock
is deleted, remove the virtual environment and reinstall all dependencies.
# Remove the current virtual environment
pipenv --rm
# Reinstall dependencies and recreate the virtual environment
pipenv install
Structure:
[packages]
and [dev-packages]
.Version Specification:
==
, >=
, or ~=
.Usage:
pip
for installing dependencies directly.Locking Dependencies:
Pipfile.lock
to lock dependencies to specific versions.pip-tools
.pip install pipenv
Pipfile
with your package configurations, including both [packages]
and [dev-packages]
sections.pipenv install
to read the Pipfile
, install the specified packages, and generate a Pipfile.lock
.
pipenv install
Pipfile
, update the lock file.
pipenv lock
Here's an example Pipfile
for a Django application:
[[source]]
url = "https://pypi.org/simple"
verify_ssl = true
name = "pypi"
[packages]
django = "==3.1.4"
celery = "*"
sentry-sdk = "==1.3.1"
python-decouple = "==3.5"
# ... other packages ...
[dev-packages]
mypy = "*"
pytest = "*"
# ... other dev packages ...
[requires]
python_version = "3.10"
[pipenv]
allow_prereleases = true
Explanation:
[[source]]
: Specifies the package source, in this case, PyPI.[packages]
: Lists the production dependencies with specific versions.[dev-packages]
: Lists development dependencies.[requires]
: Specifies the required Python version.[pipenv]
: Additional Pipenv configurations.When you install or uninstall packages using Pipenv, both the Pipfile
and Pipfile.lock
are automatically updated to reflect the changes. This ensures that your project's dependencies remain consistent and reproducible.
pipenv install
pipenv shell
python manage.py migrate
python manage.py runserver
pipenv install new_package
pipenv update
pipenv check
pipenv lock -r > requirements.txt
Pyenv is a tool that allows you to easily switch between multiple versions of Python. It's particularly useful when working on projects that require different Python versions.
pyenv install 3.9.1
pyenv global 3.9.1
pyenv local 3.8.10
pyenv shell 3.8.10
pyenv versions
Pipenv and Pyenv serve different but complementary purposes in Python development.
Pipfile
and Pipfile.lock
for dependency management.Combining Pipenv and Pyenv allows you to manage both Python versions and project dependencies effectively:
When you run pipenv install
, Pipenv determines which Python version to use through the following mechanisms:
Pipfile
.
[requires]
python_version = "3.8"
[requires]
python_version = "3.8"
pipenv install
PIPENV_PYTHON
environment variable.Pipfile
for the required Python version.Pipenv does not install Python versions. It relies on the specified Python version being already installed on your system. To automatically install and manage Python versions, you should use Pyenv in conjunction with Pipenv.
To create a workflow similar to Node.js's .nvmrc
with nvm
, follow these steps:
curl https://pyenv.run | bash
~/.bashrc
, ~/.zshrc
, etc.):
export PATH="$HOME/.pyenv/bin:$PATH"
eval "$(pyenv init --path)"
eval "$(pyenv init -)"
.python-version
file in your project directory:
echo "3.8.10" > .python-version
pyenv install 3.8.10
pyenv local 3.8.10
[requires]
python_version = "3.8"
pipenv install
You can automate the setup process with a script:
#!/bin/bash
# Ensure pyenv is installed
if ! command -v pyenv &> /dev/null; then
curl https://pyenv.run | bash
export PATH="$HOME/.pyenv/bin:$PATH"
eval "$(pyenv init --path)"
eval "$(pyenv init -)"
fi
# Specify Python version
PYTHON_VERSION="3.8.10"
# Create .python-version file
echo $PYTHON_VERSION > .python-version
# Install Python version with pyenv
pyenv install -s $PYTHON_VERSION
# Set local Python version
pyenv local $PYTHON_VERSION
# Create Pipfile if it doesn't exist
if [ ! -f "Pipfile" ]; then
pipenv --python $PYTHON_VERSION
fi
# Install dependencies
pipenv install
.python-version
file.
pyenv local 3.9.1
pyenv version
The output should display the Python version specified in .python-version
.
export PATH="$HOME/.pyenv/bin:$PATH"
eval "$(pyenv init --path)"
eval "$(pyenv init -)"
source ~/.bashrc # or source ~/.zshrc
pyenv rehash
PYENV_DEBUG=1 pyenv version
Managing Python projects effectively requires the right tools to handle dependencies and Python versions. Pipenv simplifies dependency and virtual environment management, while Pyenv allows you to install and switch between multiple Python versions. By understanding and leveraging the strengths of both tools, you can create a robust and maintainable development environment.
Key Takeaways:
Pipfile
and .python-version
files for consistency.By following the guidelines and examples provided in this guide, you'll be well-equipped to handle the complexities of Python project management, ensuring your applications are built on solid and consistent foundations.
Comments
Post a Comment