How to Install and Manage PostGIS with a Non-Superuser Role
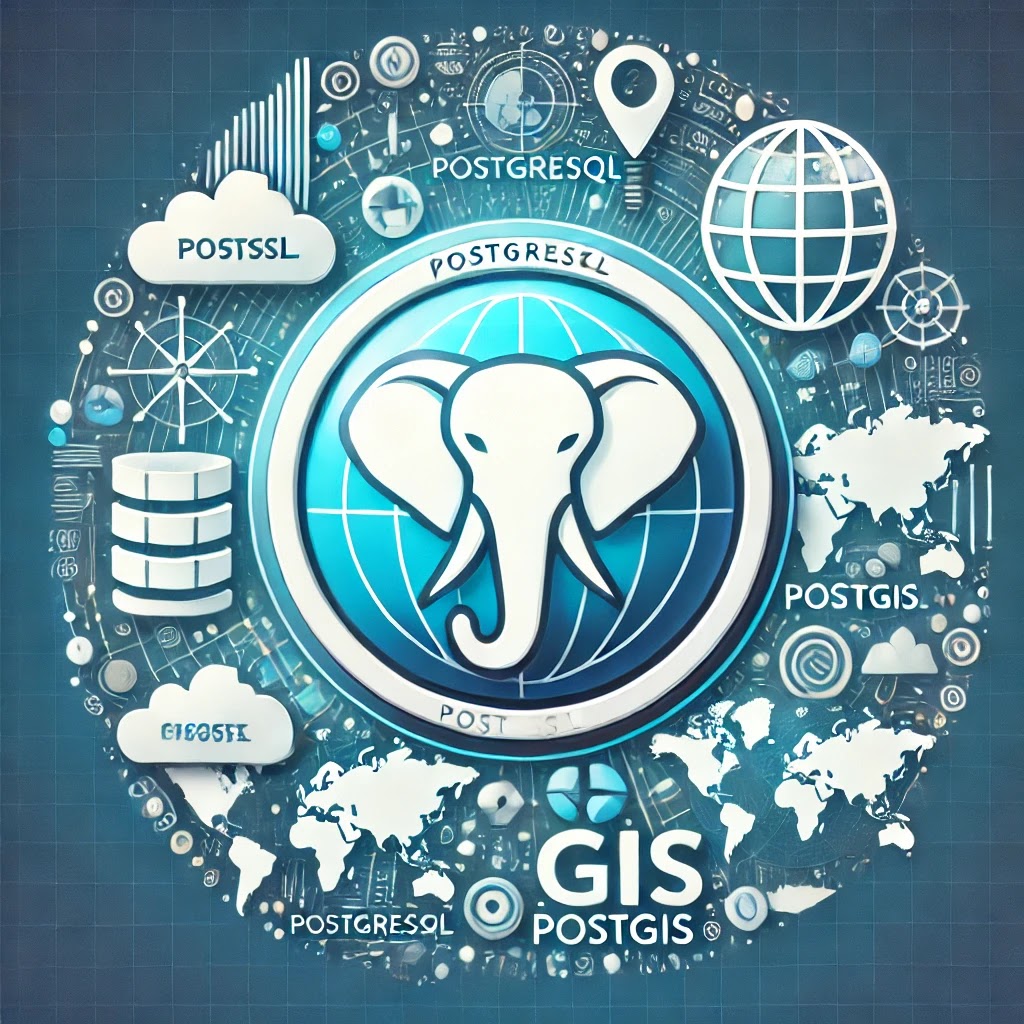
In high-traffic applications, it’s essential to protect server resources and ensure fair usage among users. Throttling is a method used in Django REST Framework (DRF) to limit the rate of requests, helping prevent abuse and manage load. In this article, we’ll explore how to implement throttling in DRF, including ways to apply it to specific views and dynamically whitelist IPs.
Throttling controls the rate of requests an API endpoint can receive from a single client. It’s a valuable tool for:
In Django REST Framework, throttling is easy to configure with built-in classes like UserRateThrottle
and AnonRateThrottle
.
To set up global throttling for all requests, define DEFAULT_THROTTLE_CLASSES
and DEFAULT_THROTTLE_RATES
in your settings.py
file:
# settings.py
REST_FRAMEWORK = {
'DEFAULT_THROTTLE_CLASSES': [
'rest_framework.throttling.UserRateThrottle',
'rest_framework.throttling.AnonRateThrottle',
],
'DEFAULT_THROTTLE_RATES': {
'user': '1000/day', # Limits authenticated users to 1000 requests per day
'anon': '100/hour', # Limits anonymous users to 100 requests per hour
}
}
If you only need throttling for certain endpoints, set throttle_classes
directly in the view:
from rest_framework.views import APIView
from rest_framework.throttling import ScopedRateThrottle
class LimitedAccessView(APIView):
throttle_classes = [ScopedRateThrottle]
throttle_scope = 'limited_access' # Reference a custom scope in settings.py
def get(self, request):
return Response({"message": "This endpoint is throttled to 10 requests per minute."})
In settings.py
, define the custom scope:
# settings.py
REST_FRAMEWORK = {
'DEFAULT_THROTTLE_RATES': {
'limited_access': '10/minute', # Custom rate for specific endpoint
}
}
For larger applications, you may want to store whitelisted IPs in a database, such as MongoDB, to allow dynamic updates. Here’s an example of using MongoDB with a custom throttle class:
from pymongo import MongoClient
from rest_framework.throttling import SimpleRateThrottle
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database_name']
whitelisted_ips_collection = db['whitelisted_ips']
class CustomIPThrottle(SimpleRateThrottle):
scope = 'custom_ip'
def get_cache_key(self, request, view):
ip_addr = self.get_ident(request)
if whitelisted_ips_collection.find_one({"ip_address": ip_addr}):
return None # Skip throttling for whitelisted IPs
return ip_addr
# settings.py
REST_FRAMEWORK = {
'DEFAULT_THROTTLE_CLASSES': [
'path.to.CustomIPThrottle', # Use the custom throttle class
],
'DEFAULT_THROTTLE_RATES': {
'custom_ip': '50/hour',
}
}
With this setup, requests from whitelisted IPs will bypass throttling, while other IPs will be limited based on the specified rate.
Throttling is a crucial part of building scalable and secure APIs. By implementing DRF’s built-in throttling classes, configuring custom throttling for specific views, and dynamically whitelisting IPs from a database, you can manage high traffic efficiently while preventing abuse. These strategies help ensure that your API remains responsive and available to legitimate users.
Throttling in Django REST Framework (DRF) is a technique used to limit the rate of requests a client can make to an API. This prevents abuse, manages server load, and ensures fair resource usage by applying rate limits on requests from individual users or IP addresses.
Throttling is essential in high-traffic applications to prevent overloading server resources, maintain performance, and reduce the impact of potential malicious attacks. By controlling the number of requests, throttling helps in preserving the availability and responsiveness of the API for all users.
Django REST Framework includes several throttle classes, such as:
UserRateThrottle
: Limits the rate of requests for authenticated users.AnonRateThrottle
: Limits the rate of requests for anonymous users.ScopedRateThrottle
: Applies specific rate limits to particular views, allowing different rates per endpoint.To set global throttling rates, define DEFAULT_THROTTLE_CLASSES
and DEFAULT_THROTTLE_RATES
in the settings.py
file. For example:
# settings.py
REST_FRAMEWORK = {
'DEFAULT_THROTTLE_CLASSES': [
'rest_framework.throttling.UserRateThrottle',
'rest_framework.throttling.AnonRateThrottle',
],
'DEFAULT_THROTTLE_RATES': {
'user': '1000/day', # Authenticated users can make 1000 requests per day
'anon': '100/hour', # Anonymous users can make 100 requests per hour
}
}
Yes, you can apply throttling to specific views by using the throttle_classes
attribute directly in the view. This allows you to set custom throttling limits on particular endpoints without affecting the rest of the API.
self.get_ident(request)
in custom throttling classes?The self.get_ident(request)
method is used to extract a unique identifier, typically the IP address, from the incoming request. This is useful in custom throttling classes where we want to apply rate limits based on the client’s IP address. If the IP address is found in the whitelist (e.g., stored in a database), throttling can be bypassed by returning None
. Otherwise, the IP address is used as the cache key to track the request rate.
def get_cache_key(self, request, view):
ip_addr = self.get_ident(request)
# Check if IP is whitelisted
if whitelisted_ips_collection.find_one({"ip_address": ip_addr}):
return None # Skip throttling for whitelisted IPs
return ip_addr
To whitelist specific IP addresses, you can create a custom throttle class that checks if an IP is in a whitelist (e.g., a database collection). If the IP is whitelisted, the custom class can return None
to skip throttling. This allows dynamic control over which IPs can bypass rate limits.
Yes, by storing whitelisted IPs in a database (e.g., MongoDB or PostgreSQL), you can update the whitelist dynamically. Each time a request is received, the custom throttle class can query the database to check if the IP is whitelisted, allowing real-time adjustments without needing to modify the code.
When a user exceeds their request limit, DRF will automatically respond with a 429 "Too Many Requests" HTTP status code. This indicates that the client should wait before making further requests.
Yes, DRF allows you to customize the throttling response by overriding the throttle_wait
method in your custom throttle class or by modifying the default exception handling. You can display custom messages or instructions on how to proceed if the request limit is reached.
Comments
Post a Comment