How to Install and Manage PostGIS with a Non-Superuser Role
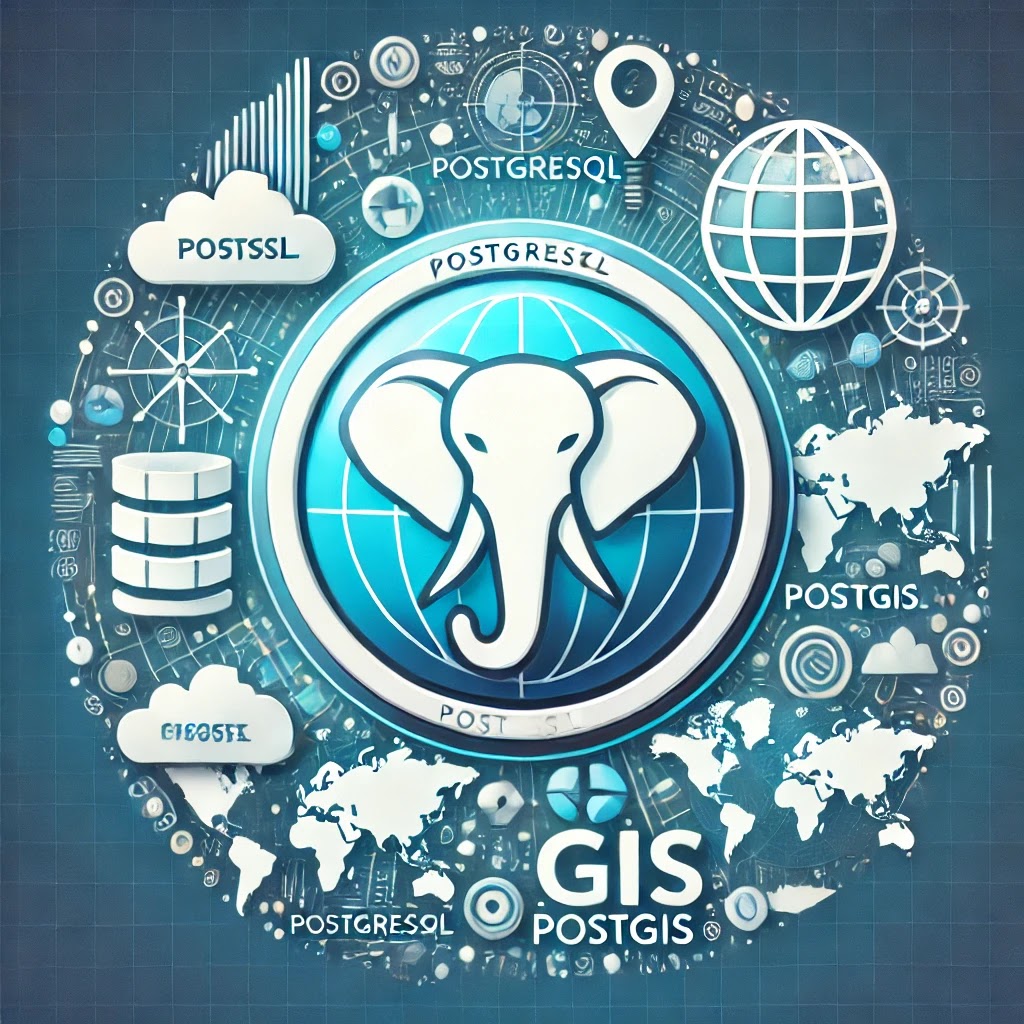
JavaScript, one of the most popular programming languages in the world, is based on the ECMAScript (ES) standard. Over the years, ECMAScript has evolved significantly, introducing new features and improvements that have shaped the modern JavaScript we use today. In this post, we'll explore the key ECMAScript versions, highlighting the most important features that have been added along the way.
The first version of ECMAScript established the basic syntax and functionality of JavaScript.
This version was a minor update, mainly for aligning with the ISO/IEC 16262 international standard, without any major new features.
This version introduced several important features that made JavaScript more powerful:
try {
// code that may throw an error
} catch (e) {
console.log(e);
}
ES5 brought several critical improvements, making JavaScript more reliable:
forEach()
, map()
, filter()
, etc.JSON.stringify()
and JSON.parse()
.
"use strict";
const arr = [1, 2, 3];
const squared = arr.map(x => x * x);
console.log(squared);
Also known as ES6 or ES2015, this version modernized JavaScript, introducing many new features:
const add = (a, b) => a + b;
const greeting = `Hello, ${name}!`;
This version introduced two notable features:
**
).
console.log([1, 2, 3].includes(2)); // true
console.log(2 ** 3); // 8
ES8 made asynchronous code easier with the introduction of async/await:
async function fetchData() {
const response = await fetch('https://api.example.com');
const data = await response.json();
console.log(data);
}
Key features of ES9 include:
for-await-of
loop.
const obj = {a: 1, b: 2, c: 3};
const {a, ...rest} = obj;
console.log(rest); // {b: 2, c: 3}
ES10 added useful features like:
catch
block no longer requires an error argument.
const arr = [1, [2, 3], [4, [5]]];
console.log(arr.flat(2)); // [1, 2, 3, 4, 5]
ES11 focused on flexibility:
const foo = null ?? 'default'; // 'default'
console.log(user?.profile?.age); // undefined
ES12 introduced logical assignment operators, such as &&=
, ||=
, and ??=
.
let a = true;
a &&= false; // a = false
This version allows the use of await
outside async functions, making asynchronous code in modules simpler.
// Usage in modules
const data = await fetch('https://api.example.com');
console.log(data);
Comments
Post a Comment