How to Install and Manage PostGIS with a Non-Superuser Role
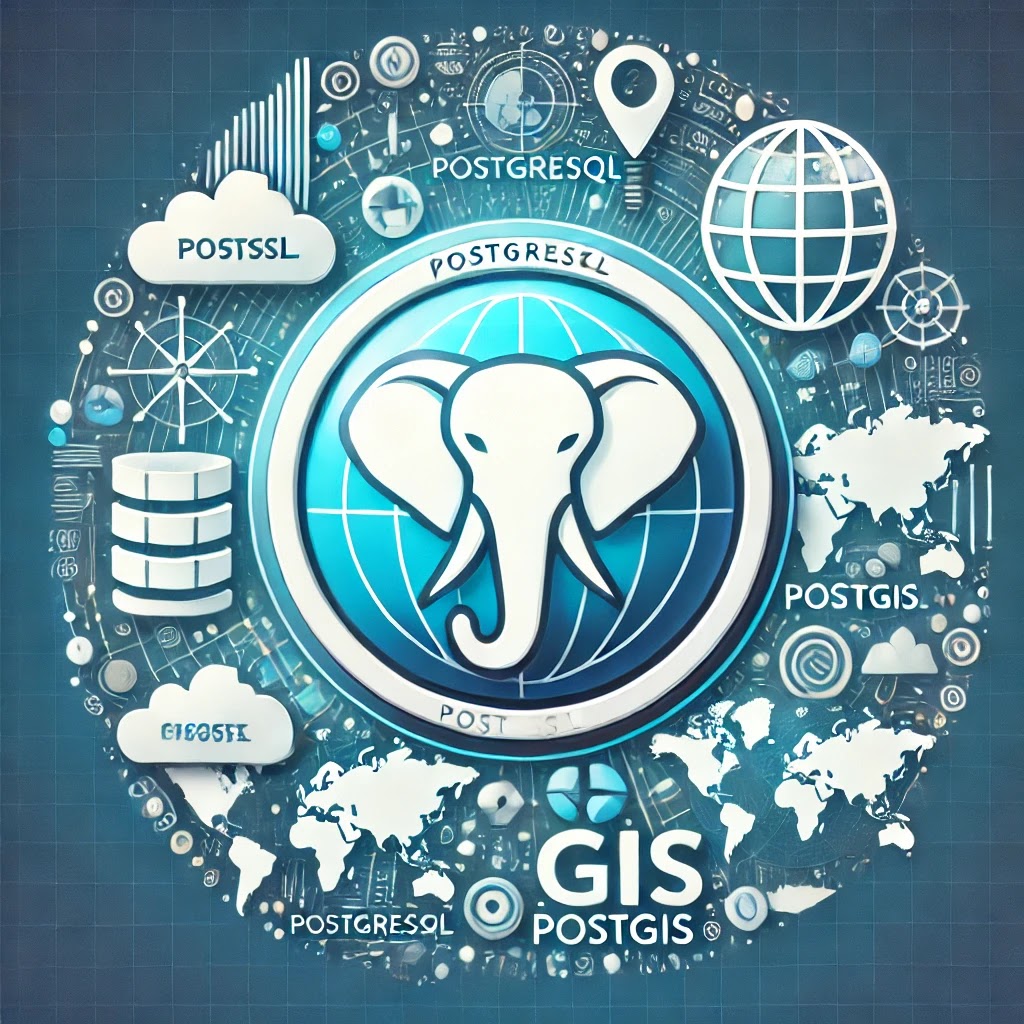
The Fibonacci sequence is one of the simplest and most fascinating concepts in mathematics. Its presence in nature, computer algorithms, and problem-solving makes it an ideal case study for understanding various programming concepts. In this blog, we will explore Fibonacci from both a mathematical and a developer's standpoint, covering recursive vs iterative approaches, the importance of time and space complexity, and the implications of shallow vs deep copying along with the behavior of primitives and objects.
The Fibonacci sequence starts with 0 and 1, with each subsequent number being the sum of the two preceding ones. Mathematically, this is expressed as:
F(n) = \begin{cases} 0 & \text{if } n = 0, \\ 1 & \text{if } n = 1, \\ F(n-1) + F(n-2) & \text{if } n \geq 2 \end{cases}
A famous closed-form formula for the Fibonacci sequence is called Binet’s formula, which can be used to find the nth Fibonacci number without recursion or iteration:
\[ F_n = \frac{1}{\sqrt{5}} \left( \left(\frac{1 + \sqrt{5}}{2}\right)^n - \left(\frac{1 - \sqrt{5}}{2}\right)^n \right) \]
Where \( \phi = \frac{1 + \sqrt{5}}{2} \) is the golden ratio.
The recursive Fibonacci function closely mirrors the mathematical definition of the sequence. In programming, this can be represented as:
// Recursive Fibonacci function with memoization
function recursiveFibonacci(number) {
// Ensure the number is a valid integer
number = parseInt(number);
let series = []; // Array to store Fibonacci series
// Check if the number is valid
if (number <= 0 || isNaN(number)) {
return 'Enter a positive number';
}
let memory = {}; // Memory object to store previously computed values
// Inner recursive function for Fibonacci calculation
function fibonacci(n) {
// Check if the value is already in memory
if (n in memory) {
return memory[n];
}
// Base cases
if (n === 1) return 0;
if (n === 2) return 1;
// Recursive case for n >= 3
let result = fibonacci(n - 1) + fibonacci(n - 2);
// Store the result in memory for future use
memory[n] = result;
return result;
}
// Generate Fibonacci series up to the given 'number'
for (let i = 1; i <= number; i++) {
let fibNumber = fibonacci(i);
series.push(fibNumber);
}
return series;
}
// Example usage:
console.log(recursiveFibonacci(6));
def fibonacci_series(n):
def fibonacci(num):
if num <= 0:
return "Input should be a positive integer"
elif num == 1:
return 0
elif num == 2:
return 1
else:
return fibonacci(num - 1) + fibonacci(num - 2)
series = []
for i in range(1, n + 1):
series.append(fibonacci(i))
return series
# Example usage:
n = 10
print(f"Fibonacci series till {n}:", fibonacci_series(n))
The time complexity of the recursive Fibonacci function is determined by the number of function calls and how many times each value is computed.
O(2^n)
, due to
the exponential number of calls.memory
object.
Thus, the function computes each Fibonacci number exactly once.Therefore, the overall time complexity with memoization is:
Time complexity: O(n)
This is because the function only needs to compute each Fibonacci number once, and subsequent lookups are in
constant time, O(1)
, due to the memoization technique.
The space complexity is determined by two factors:
n
, this
contributes O(n)
to the space complexity.memory
object stores each Fibonacci number, which also takes
O(n)
space.
series
array also stores the Fibonacci sequence, contributing another O(n)
.
Thus, the total space complexity is:
Space complexity: O(n)
The use of memoization greatly optimizes the recursive Fibonacci function, reducing both the time and space
complexities to O(n)
.
This allows us to calculate Fibonacci numbers efficiently even for larger values of n
.
Although this approach mirrors the math definition, it is inefficient for large inputs because it recalculates the same values multiple times.
The time complexity of the recursive Fibonacci function without memoization is O(2^n)
because each call to the function spawns two more calls, forming a binary tree of calls with exponential growth.
Consider the Fibonacci function defined as:
F(n) = F(n-1) + F(n-2)
With base cases:
F(0) = 0 and F(1) = 1
If we call Fibonacci(n)
, the function recursively calls Fibonacci(n-1)
and Fibonacci(n-2)
. This pattern repeats for each subsequent call, forming a binary tree where each node represents a function call.
For example, for Fibonacci(5)
:
F(5)
/ \
F(4) F(3)
/ \ / \
F(3) F(2) F(2) F(1)
/ \ / \ / \
F(2) F(1) F(1) F(0) F(1) F(0)
As seen in the tree:
F(5)
calls F(4)
and F(3)
.F(4)
calls F(3)
and F(2)
.In this tree, each node (function call) spawns two more nodes (function calls) until reaching the base cases.
n
.F(n)
)F(n-1)
and F(n-2)
)This pattern continues, and the number of calls per level grows exponentially as 2^k
, where k
is the level.
Since the tree grows exponentially and has a depth of n
, the total number of function calls is approximately 2^n
. This results in the time complexity of O(2^n)
.
This inefficiency arises because each call recalculates the same values multiple times. For example, F(3)
and F(2)
are computed multiple times, leading to redundant work.
An iterative approach is more efficient because it avoids redundant calculations. Here's the iterative approach:
function generateFibonacci(n) {
if (n <= 0) return [];
if (n === 1) return [0];
let fib = [0, 1];
let a = 0, b = 1;
for (let i = 2; i < n; i++) {
let next = a + b;
a = b;
b = next;
fib.push(next);
}
return fib;
}
// Example: Generate first 10 Fibonacci numbers
console.log(generateFibonacci(10));
def generate_fibonacci(n):
if n <= 0:
return []
if n == 1:
return [0]
fib = [0, 1]
a, b = 0, 1
for i in range(2, n):
next_num = a + b
a = b
b = next_num
fib.append(next_num)
return fib
# Example: Generate first 10 Fibonacci numbers
print(generate_fibonacci(10))
The iterative approach runs in O(n) time and uses O(1) space, making it far more efficient than the recursive approach.
Time complexity describes how the runtime of the algorithm scales with the input size, while space complexity describes how much memory is required.
When dealing with arrays or objects in programming, it's important to understand the difference between shallow copies and deep copies.
let fibonacciArray = [0, 1, 1, 2, 3, 5];
let shallowCopy = fibonacciArray.slice();
shallowCopy[0] = 10;
console.log(fibonacciArray); // [0, 1, 1, 2, 3, 5]
console.log(shallowCopy); // [10, 1, 1, 2, 3, 5]
let complexArray = [0, { a: 1 }];
let deepCopy = JSON.parse(JSON.stringify(complexArray));
deepCopy[1].a = 10;
console.log(complexArray[1].a); // 1 (original object remains unchanged)
console.log(deepCopy[1].a); // 10
Primitives (numbers, strings, booleans) are copied by value.
let a = 5;
let b = a;
b = 10;
console.log(a); // 5
Objects and Arrays are copied by reference.
let obj = { val: 10 };
let ref = obj;
ref.val = 20;
console.log(obj.val); // 20 (both refer to the same object)
The Fibonacci sequence is not just a mathematical curiosity but a window into important programming concepts like recursion, iteration, and performance optimization. By exploring both the mathematical definition and the implementation in code, developers can gain a deeper understanding of how to approach problems and write efficient algorithms.
https://study.com/academy/lesson/what-is-the-golden-ratio-in-math-definition-examples.html
ReplyDelete