How to Install and Manage PostGIS with a Non-Superuser Role
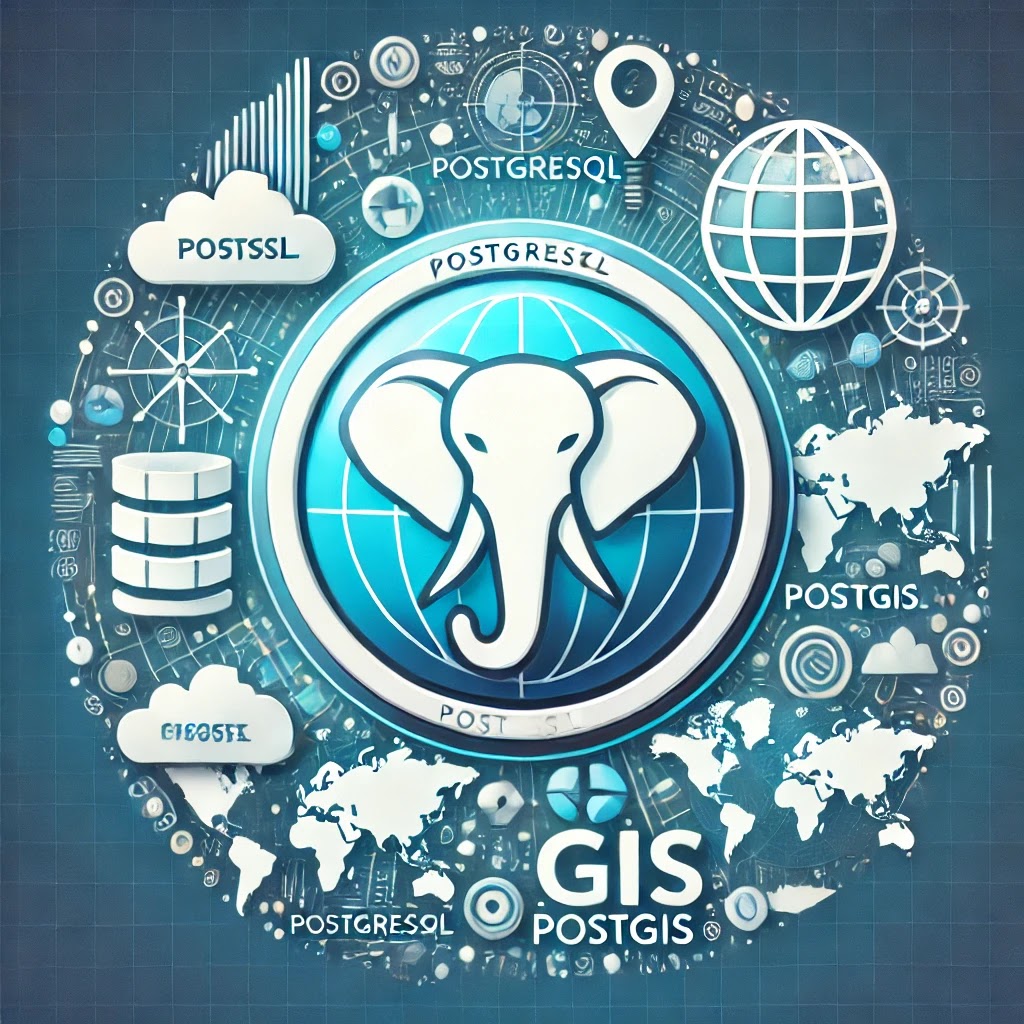
Prerequisite: PostgreSQL Version This guide assumes you are using PostgreSQL version 14 or later, which supports the necessary commands for PostGIS installation and management. Ensure your PostgreSQL server is up-to-date before proceeding. This guide ensures that PostGIS is installed and configured properly for a specific user, such as administrator , while avoiding common issues. 1. Ensure Superuser Access sudo -i -u postgres psql --dbname=financethat 2. Create a Role for PostGIS Management CREATE ROLE administrator WITH LOGIN PASSWORD 'your_secure_password'; GRANT ALL PRIVILEGES ON DATABASE financethat TO administrator; 3. Install PostGIS To install PostGIS on Ubuntu, first ensure you have the required PostgreSQL version installed. Then, use the following commands: sudo apt update sudo apt install postgis postgresql-14-postgis-3 Replace 14 with your PostgreSQL version if different. After installation, enable PostGIS in...